Arduino has long been celebrated for its versatility in creating DIY electronics projects. In this guide, we’ll explore how you can leverage Arduino Ethernet Shield for various networking and automation tasks, including using it as a web server, FTP server, network SD card reader, and home automation hub with wireless sensors. With these projects, we can take take the home automation and IoT capabilities of the arduino to the next level.
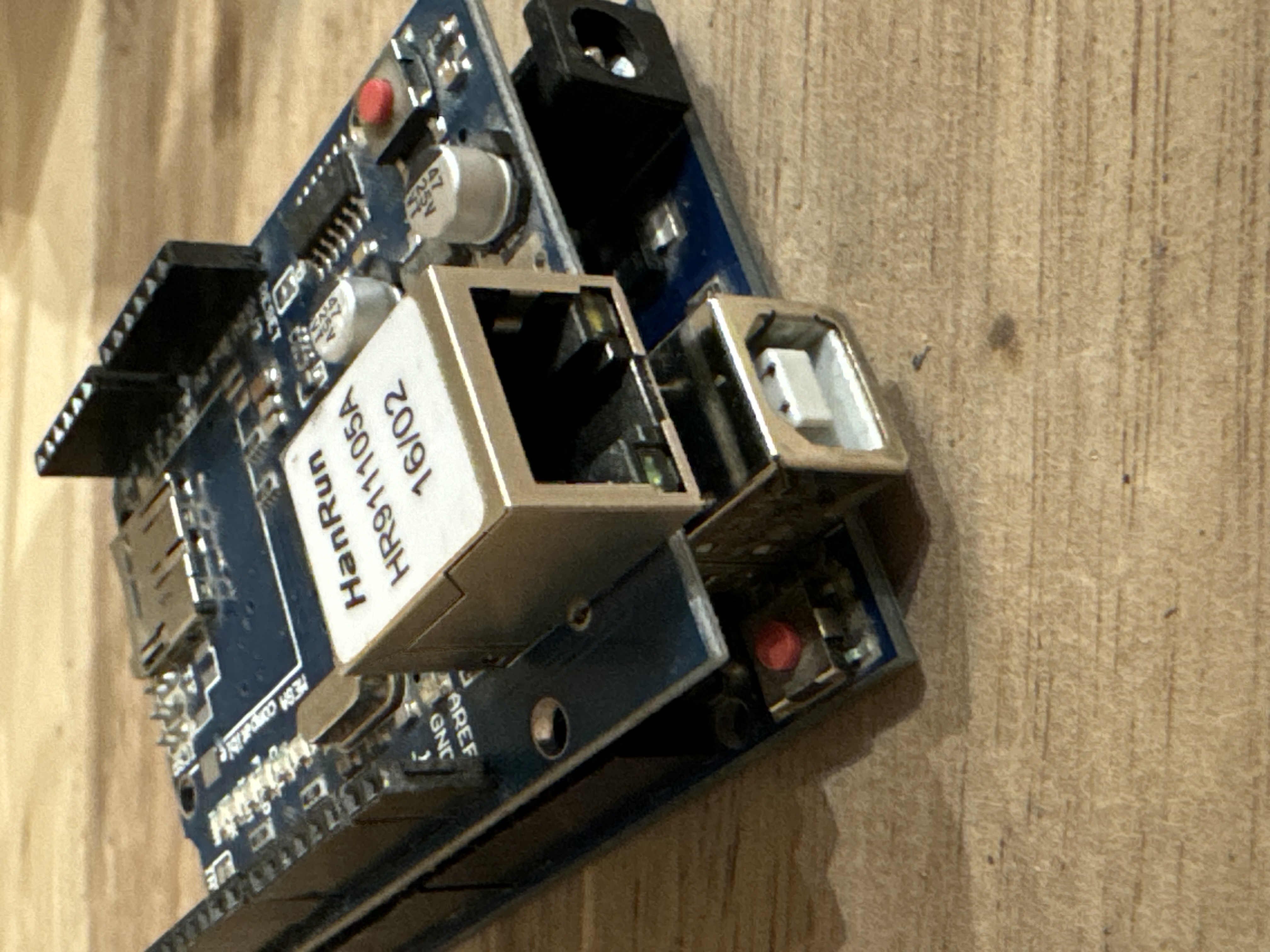
What You’ll Need:
- Arduino Uno – The brain of the project.
- Ethernet Shield (W5100-based) – This shield provides SD card functionality as well as Ethernet capabilities.
- SD Card – Make sure it’s formatted properly (FAT16 or FAT32).
- USB Adapter – To connect your SD card to your computer for testing or transferring files.
- USB to Type B Cable – Used to upload code from your computer to the Arduino Uno.
- USB Power Adapter – To power the Arduino externally if needed.
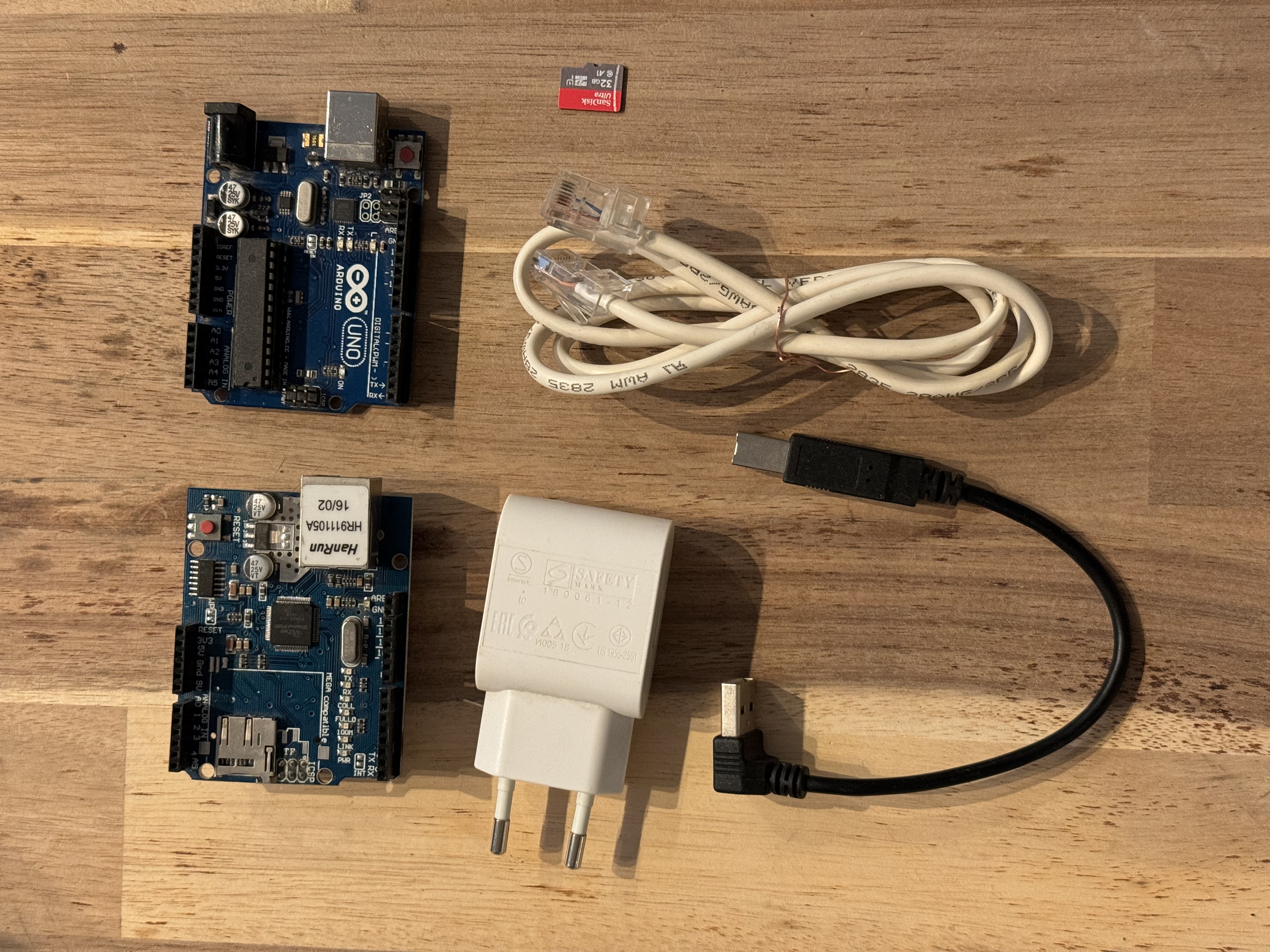
Understanding the W5100 Network Chip
The Ethernet Shield used in this project is based on the W5100 network chip, which provides both Ethernet capabilities and SD card interfacing. The W5100 is a full-featured, single-chip internet connectivity solution for embedded systems. It supports both IPv4 and TCP/IP, meaning you can use it to connect your Arduino to a network, and, in our case, interact with an SD card.
Key features of the W5100 include:
- Supports 10/100 Ethernet: While we won’t use Ethernet in this specific project, it's good to know that the chip is capable of handling networking tasks as well.
- Built-in SD Card Interface: The SD card slot on the Ethernet Shield is directly managed by the W5100, allowing you to easily read from or write to an SD card.
- SPI Interface: The W5100 communicates with the Arduino via the Serial Peripheral Interface (SPI), which is ideal for fast data transfer.
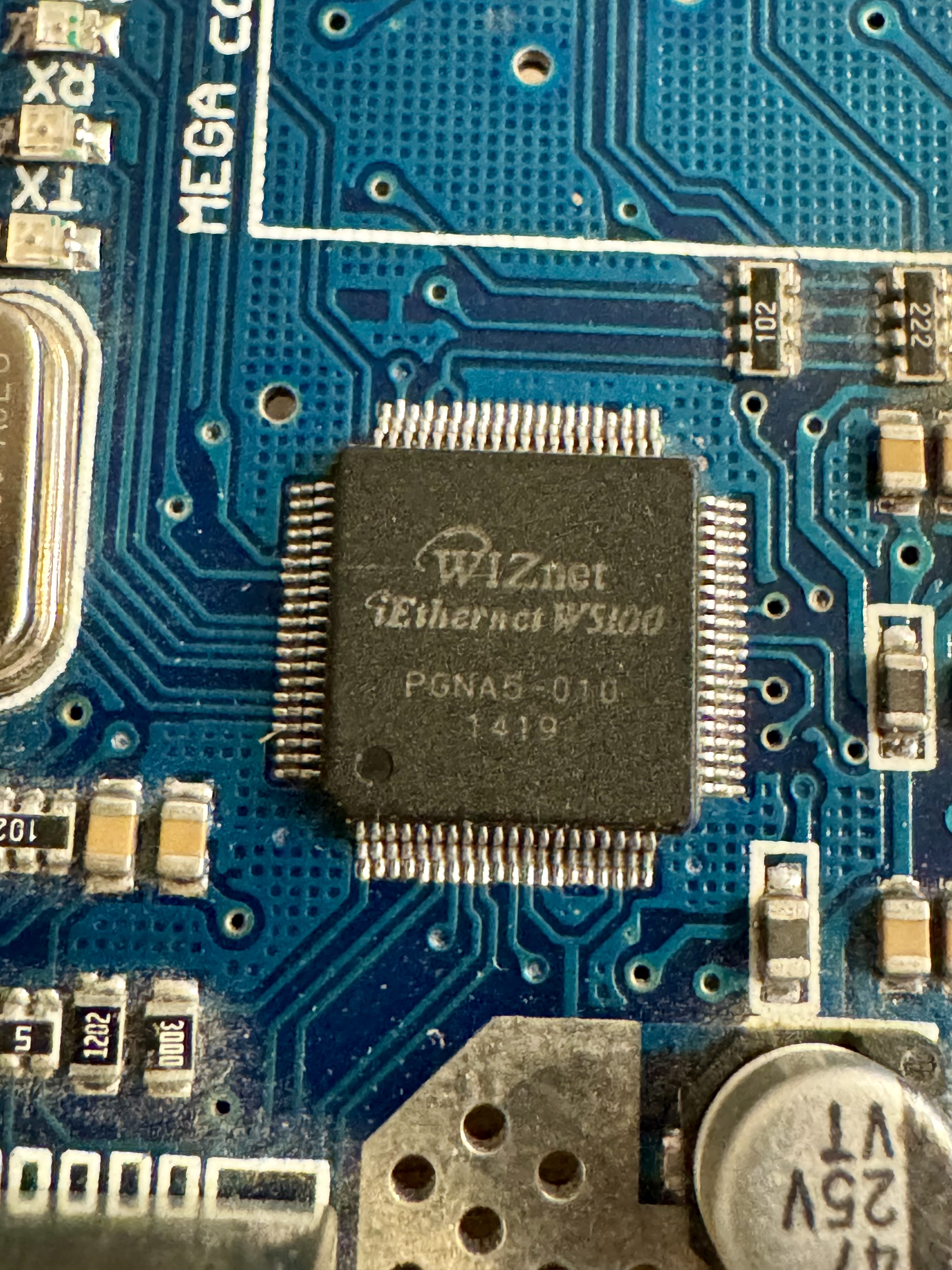
Network Test for Ethernet Shield
This section will guide you through testing the network connection of an Arduino with an Ethernet Shield. We will use the Ethernet library to perform a basic test that verifies if the Arduino is able to obtain an IP address and connect to a server or a local network.
Step 1: Connect the Ethernet Shield
- Attach the Ethernet shield on top of your Arduino board.
- Connect an Ethernet cable to the Ethernet port of the shield.
- Make sure the other end of the cable is connected to your router or a switch on the same network.
Step 2: Upload the Test Code
Open the Arduino IDE and upload the following code to your Arduino. This code will attempt to connect to your local network and display the IP address assigned to the Ethernet shield.
#include <SPI.h>
#include <Ethernet.h>
// MAC address for your Ethernet Shield (find on the sticker on the back)
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
// Initialize the Ethernet client library
// with the IP address and port of the server (port 80 is default for HTTP):
EthernetClient client;
void setup() {
// Open serial communications and wait for the port to open:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
// Start the Ethernet connection:
Serial.println("Initializing Ethernet...");
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to configure Ethernet using DHCP");
// No point in carrying on, so do nothing forevermore:
while (true);
}
// Give the Ethernet shield a second to initialize:
delay(1000);
// Print the local IP address:
Serial.print("My IP address: ");
Serial.println(Ethernet.localIP());
}
void loop() {
// Your network code here (if needed for further testing)
}
Step 3: Monitor the Serial Output
- Open the Serial Monitor in the Arduino IDE (Tools > Serial Monitor) and set the baud rate to
9600
. - Once the code runs, the Serial Monitor will display the assigned IP address or an error if the connection fails.
- If you see the message "My IP address: xxx.xxx.xxx.xxx", where
xxx.xxx.xxx.xxx
is your local IP address, then your Ethernet shield is successfully connected to the network.
Step 4: Test Network Connectivity
Now, let's extend the test by connecting to a web server. You can try accessing a simple webpage or a network service. Modify the code below to connect to a website or IP address of your choice:
#include <SPI.h>
#include <Ethernet.h>
// MAC address for your Ethernet Shield
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
// Server to connect to
char server[] = "www.example.com"; // Change this to a valid server
EthernetClient client;
void setup() {
Serial.begin(9600);
// Start Ethernet and request IP from DHCP
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to configure Ethernet using DHCP");
while (true);
}
delay(1000); // Allow time for Ethernet shield to initialize
// Print IP Address
Serial.print("My IP address: ");
Serial.println(Ethernet.localIP());
// Connect to server
Serial.print("Connecting to ");
Serial.println(server);
if (client.connect(server, 80)) {
Serial.println("Connected!");
// Make a HTTP GET request:
client.println("GET / HTTP/1.1");
client.println("Host: www.example.com");
client.println("Connection: close");
client.println();
} else {
Serial.println("Connection failed.");
}
}
void loop() {
// If there are incoming bytes available from the server, read and print them:
if (client.available()) {
char c = client.read();
Serial.print(c);
}
// If the server has disconnected, stop the client:
if (!client.connected()) {
Serial.println();
Serial.println("Disconnecting.");
client.stop();
// Do nothing forevermore:
while (true);
}
}
Step 5: Check the Serial Monitor
Once the code is running, check the Serial Monitor. It should display the following:
- The IP address assigned to the Ethernet shield.
- A message indicating that it is attempting to connect to the server.
- If successful, it will display the server's response.
- If the connection fails, it will show "Connection failed".
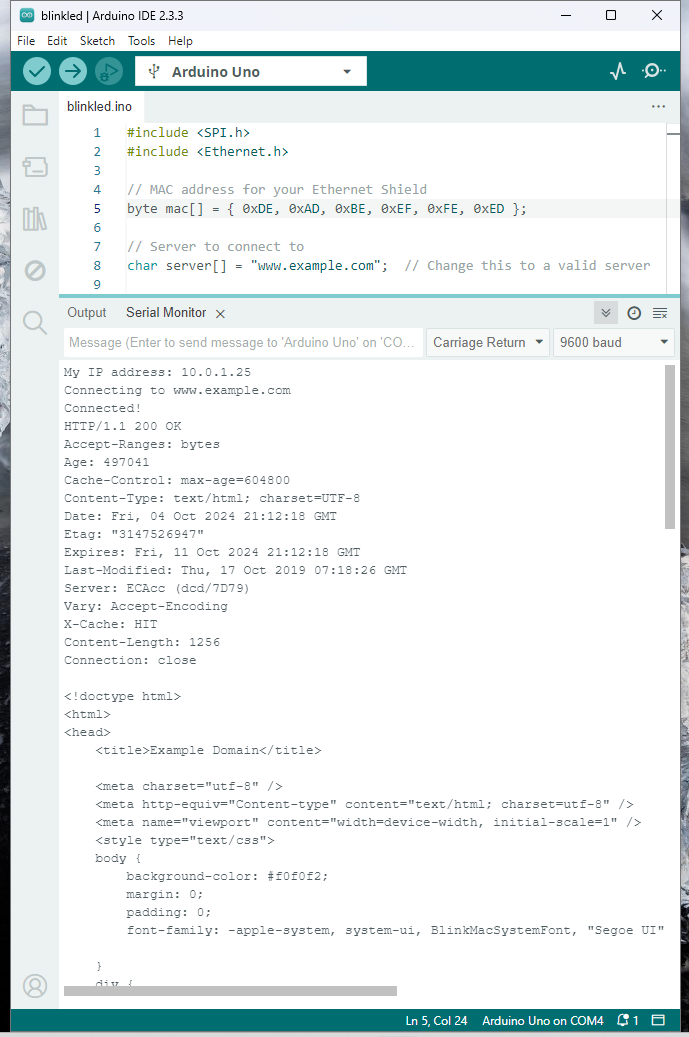
Troubleshooting Tips
- Ensure the Ethernet cable is securely connected to both the Ethernet Shield and your router.
- Verify the server address in the code is correct and reachable from your network.
- Check the MAC address is correct. You can find it on the Ethernet Shield's sticker.
- If DHCP fails, try setting a static IP address in the code.
Adding File Read and Write Functionality:
Once your SD card is detected, you can start reading from and writing to it. Here’s how you can expand the basic code to write a file to the SD card and then read it back.
Writing to the SD Card:

File myFile;
void setup() {
Serial.begin(9600);
while (!Serial) {
; // Wait for Serial port to connect.
}
if (!SD.begin(chipSelect)) {
Serial.println("SD initialization failed!");
return;
}
Serial.println("SD initialization successful.");
// Open file for writing
myFile = SD.open("test.txt", FILE_WRITE);
// Write to the file
if (myFile) {
Serial.println("Writing to test.txt...");
myFile.println("Hello, Arduino SD!");
myFile.close();
Serial.println("Writing done.");
} else {
Serial.println("Error opening file.");
}
}
Reading from the SD Card:

void loop() {
// Open file for reading
myFile = SD.open("test.txt");
if (myFile) {
Serial.println("Reading from test.txt:");
while (myFile.available()) {
Serial.write(myFile.read());
}
myFile.close();
} else {
Serial.println("Error opening file.");
}
delay(5000);
}
Now that the SD card functionality has been successfully tested, we are ready to move on to the next step! With a solid foundation in place, you can confidently proceed to setting up more advanced projects, such as creating a web server, logging sensor data, or storing configuration files. The groundwork is complete, so let's continue building more advanced Arduino applications that require file storage.
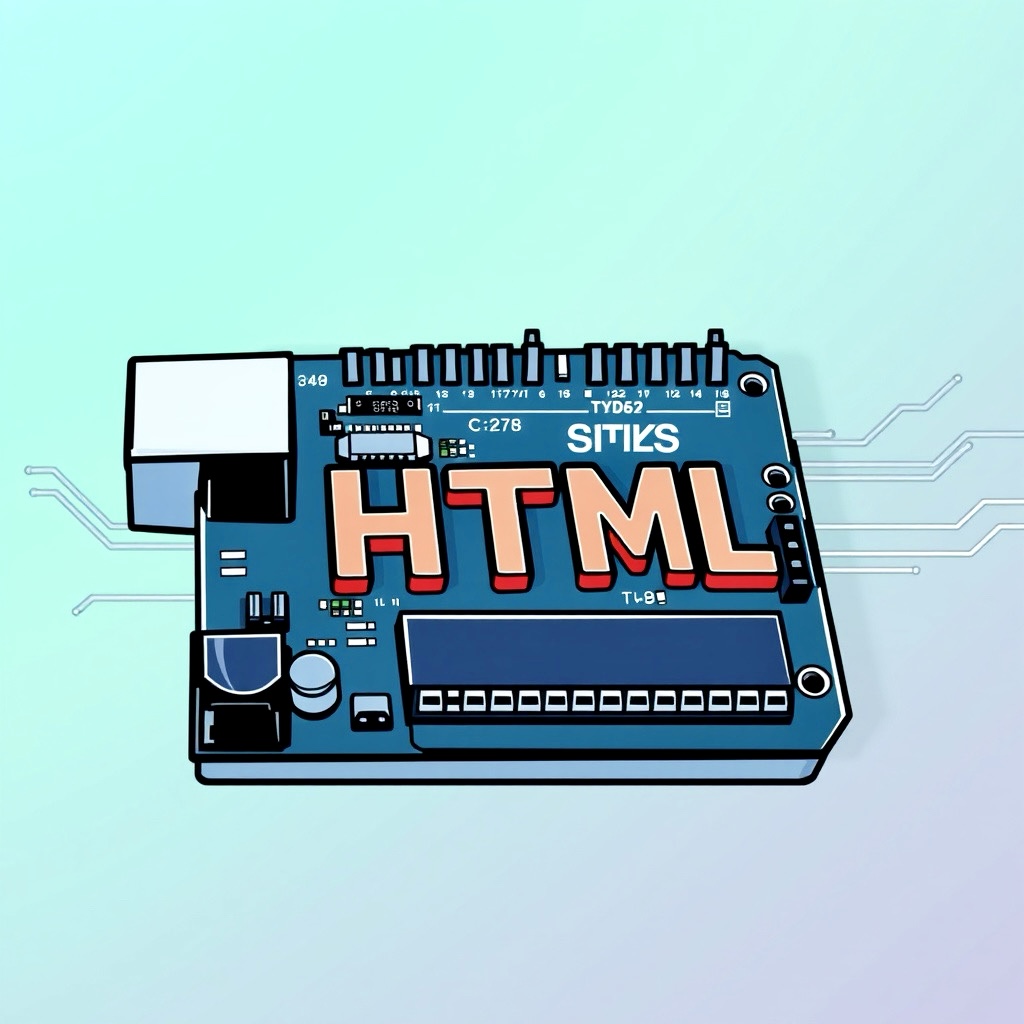
Arduino as a Web Server with Ethernet Shield
Using an Ethernet Shield, you can easily transform your Arduino into a web server. This enables you to host a webpage that can control devices such as LEDs or monitor sensors. Follow this simple guide to set up your Arduino as a web server and access it from anywhere.
Hardware Requirements
- Arduino Uno (or any compatible Arduino model)
- Ethernet Shield
- Ethernet Cable
- LEDs or Sensors (optional)
Step 1: Connect the Ethernet Shield
First, attach the Ethernet Shield to your Arduino. Plug the Ethernet cable into the shield and connect the other end to your router.
Step 2: Write the Arduino Code
Next, upload the following sketch to your Arduino. This code sets up the web server on your Arduino and responds to incoming HTTP requests with a simple webpage.
// Include Ethernet library
#include
#include
// MAC address for Ethernet Shield
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
// Set the IP address of the Arduino (modify if necessary)
IPAddress ip(192, 168, 1, 177);
// Create an Ethernet server on port 80
EthernetServer server(80);
void setup() {
// Start serial communication for debugging
Serial.begin(9600);
// Initialize the Ethernet device
Ethernet.begin(mac, ip);
server.begin();
Serial.println("Server is ready. IP address: ");
Serial.println(Ethernet.localIP());
}
void loop() {
// Listen for incoming clients
EthernetClient client = server.available();
if (client) {
Serial.println("Client connected");
// Read the HTTP request from the client
String request = client.readStringUntil('\r');
Serial.println(request);
// Prepare and send the response
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println();
client.println("");
client.println("Arduino Web Server ");
client.println("Arduino Web Server
");
client.println("Control LEDs or read sensor data here.
");
client.println("");
client.stop(); // Close the connection
Serial.println("Client disconnected");
}
}
Step 3: Accessing the Web Page Locally
Once the code is uploaded, open your browser and type in the IP address of your Arduino (e.g., http://192.168.1.177
). This will display the simple webpage hosted by your Arduino.
Step 4: Accessing the Web Page Globally
To access your web server from outside your home network, you need to configure port forwarding on your router. Here’s how:
- Log into your router's admin page by entering its IP address in your browser (usually
192.168.1.1
). - Go to the port forwarding section.
- Forward port
80
(or the port your server is running on) to the local IP of your Arduino (e.g.,192.168.1.177
). - After saving the settings, you can access your Arduino web server by entering your public IP followed by the port number (e.g.,
http://your-public-ip:80
).
You can find your public IP by searching "What is my IP" on Google.
Step 5: Optional - Using a Domain Name
For easier access, you can set up a domain name using a Dynamic DNS (DDNS) service like No-IP or DynDNS. This will allow you to access your web server using a user-friendly URL (e.g., http://yourdomain.ddns.net
).
Conclusion
With this setup, you now have an Arduino web server that can be accessed both locally and globally. This is ideal for remote monitoring, controlling devices, or managing smart home systems from anywhere.

Arduino as an FTP Server
With an Ethernet Shield or Wi-Fi module, you can also transform your Arduino into an FTP server, allowing you to upload or download files from an SD card remotely. This setup is useful for projects where data logging or file management is needed.
- Setup: Use an SD card reader on the Ethernet Shield to store files, and set up FTP functionality with libraries like ArduinoFTPServer.
- Use Case: This is ideal for uploading configuration files, sensor data logs, or even images captured from a connected camera.

Arduino as a Network SD Card Reader
By combining Arduino with an SD card reader and network functionality, you can create a basic network storage device. This enables you to access files on an SD card over your local network.
Here’s how you can do it:
- Hardware: Arduino Uno, Ethernet Shield with SD card slot.
- Code: Use the SD and Ethernet libraries to create a file server that serves files stored
- Hardware: Arduino Uno, Ethernet Shield with SD card slot.
- Code: Use the SD and Ethernet libraries to create a file server that serves files stored on the SD card over the network. You can also build an interface that allows users to upload or download files via a browser.
- Access: Access the SD card through the Arduino's IP address and navigate the file system on your browser, just like with any network-attached storage device.
This setup can be useful for sharing sensor data logs or uploading firmware updates to devices on your network.
Arduino for Home Automation
Arduino is an excellent platform for building your own home automation system. Whether it's controlling lights, monitoring temperature, or automating window blinds, the possibilities are endless. By integrating sensors and relays, you can control and monitor devices via a web interface or through voice assistants like Alexa.
Popular Home Automation Projects:
- Smart Lighting: Control lights through a web interface or automate them based on sensor data such as motion or ambient light levels.
- Temperature Control: Use temperature sensors and relays to control heating and cooling systems based on real-time data.
- Security Systems: Set up motion detectors and cameras to monitor your home and trigger alarms or notifications when suspicious activity is detected.
With wireless sensors and web-based control, you can manage your smart home remotely, making it more efficient and convenient.

Wireless Sensors for Arduino
Wireless sensors can communicate with your Arduino over Wi-Fi, RF (Radio Frequency), or Bluetooth, providing real-time data for your home automation or monitoring projects. For instance, you can build a network of temperature, humidity, or motion sensors around your home that report data back to a central Arduino unit.
- Wi-Fi Sensors: Use sensors like the ESP8266 to collect data wirelessly and send it to your Arduino for processing.
- 433 MHz RF Sensors: These sensors are great for low-power, long-range data transmission, ideal for monitoring outdoor conditions.
- Bluetooth Sensors: Pair your Arduino with nearby Bluetooth devices for short-range wireless communication, perfect for mobile applications.
Wireless sensors allow for flexibility and scalability in your IoT or home automation projects, enabling you to monitor and control various systems without extensive wiring.

Arduino as a File Server
By combining Arduino's networking capabilities with its ability to interface with an SD card, you can create a simple file server. This allows you to upload and download files over the network, making Arduino a low-cost, low-power alternative for a basic file storage solution.
Key Features of an Arduino File Server:
- File Management: Store sensor logs, configuration files, or even images from connected cameras on an SD card.
- File Access: Use a web browser to browse and download files, or upload new files to the server through a simple web interface.
- Lightweight: While not as powerful as traditional file servers, Arduino provides a lightweight solution for small-scale file management tasks.
This project is particularly useful for managing files in embedded systems or remote monitoring setups where traditional servers may not be practical.

How to Upload Code to Arduino Uno Over Ethernet
This tutorial explains how to upload Arduino code to your Arduino Uno using an Ethernet shield and a web interface. While the Arduino Uno doesn't support Over-The-Air (OTA) updates natively, you can still upload code over the network using an Ethernet connection and the Arduino CLI.
Why Upload Over Ethernet?
- Convenience: Avoid connecting the Arduino to your computer every time you need to update the code.
- Remote Updates: Perform updates even when your Arduino is far from your workspace, as long as it's connected to your network.
- Efficiency: Streamlines code updates, especially when multiple Arduinos are deployed remotely.
Steps to Set Up Ethernet Firmware Upload
- Prepare your Arduino Uno with an Ethernet shield: Make sure your Arduino Uno is equipped with an Ethernet shield to allow it to connect to your local network.
- Set up a local web server: You will need a local server that can handle file uploads and execute commands using the Arduino CLI to upload the code to the Arduino over Ethernet.
Step 1: Upload Basic Sketch to Arduino Uno
First, upload a basic sketch to the Arduino Uno that uses the Ethernet shield to connect to the network. This will allow communication with your server later.
#include <SPI.h>
#include <Ethernet.h>
// Assign a MAC address for your controller.
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
// Assign a static IP address for your Arduino.
IPAddress ip(192, 168, 1, 177);
void setup() {
// Start the Ethernet connection.
Ethernet.begin(mac, ip);
// Begin Serial communication for debugging.
Serial.begin(9600);
while (!Serial) {
; // Wait for serial port to connect. Needed for Leonardo only.
}
Serial.println("Ethernet setup complete");
}
void loop() {
// Main code for your project.
}
Step 2: Set Up a Web Server
Make sure your web server (e.g., Apache or Nginx) is set up with PHP support. You'll place the following HTML and PHP files in your server's document root (e.g., /var/www/html
).
Step 3: Create HTML Form for Code Upload
Create a file named index.html
in your server directory with the following content:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Upload Code to Arduino</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
input[type="file"] {
margin: 10px 0;
}
button {
padding: 10px 20px;
font-size: 16px;
}
</style>
</head>
<body>
<h1>Upload Code to Arduino</h1>
<form id="uploadForm" action="upload.php" method="post" enctype="multipart/form-data">
<input type="file" name="arduino_code" accept=".ino" required>
<button type="submit">Upload Code</button>
</form>
<div id="status"></div>
<script>
const form = document.getElementById('uploadForm');
form.onsubmit = function(e) {
e.preventDefault(); // Prevent the default form submission
const formData = new FormData(form);
fetch('upload.php', {
method: 'POST',
body: formData
})
.then(response => response.text())
.then(data => {
document.getElementById('status').innerText = data;
})
.catch(error => {
console.error('Error:', error);
document.getElementById('status').innerText = 'Error uploading code.';
});
};
</script>
</body>
</html>
Step 4: Create PHP Script to Handle Upload
Create a file named upload.php
in the same directory with the following content:
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
if (isset($_FILES['arduino_code']) && $_FILES['arduino_code']['error'] == 0) {
$fileTmpPath = $_FILES['arduino_code']['tmp_name'];
$fileName = $_FILES['arduino_code']['name'];
$fileSize = $_FILES['arduino_code']['size'];
$fileType = $_FILES['arduino_code']['type'];
$allowedTypes = ['text/plain', 'application/octet-stream', 'application/x-arduino'];
if (!in_array($fileType, $allowedTypes)) {
echo "Error: Invalid file type.";
exit;
}
$uploadFileDir = './uploads/';
$dest_path = $uploadFileDir . $fileName;
if (move_uploaded_file($fileTmpPath, $dest_path)) {
echo "Code uploaded successfully: " . $fileName;
// Optionally call the Arduino CLI to upload the code to the Arduino Uno over Ethernet.
// shell_exec("arduino-cli upload -p /dev/ttyUSB0 --fqbn arduino:avr:uno $dest_path");
} else {
echo "Error moving the uploaded file.";
}
} else {
echo "Error: " . $_FILES['arduino_code']['error'];
}
} else {
echo "Invalid request method.";
}
?>
Step 5: Set Up the Upload Directory
Create an uploads
directory in your web server document root to store uploaded Arduino code files:
mkdir /var/www/html/uploads sudo chown -R www-data:www-data /var/www/html/uploads
Step 6: Invoke Arduino CLI
To upload the code to the Arduino after it's uploaded to the server, use the Arduino CLI to upload the .ino file over Ethernet:
shell_exec("arduino-cli upload -p /dev/ttyUSB0 --fqbn arduino:avr $dest_path");
Step 7: Access the Web Interface
Navigate to your web server's IP address or domain name (e.g., http://your-server-ip/index.html
) and use the upload form to upload Arduino code to the Uno.
Important Notes
- Ensure your Arduino CLI is properly configured with the correct board and port settings.
- For security, implement user authentication in a production environment.
- Test the process thoroughly before deploying.
Conclusion
Arduino is an incredibly versatile platform, and its ability to serve as a web server, FTP server, network SD card reader, and home automation hub proves its potential for IoT and automation projects. By integrating wireless sensors, Ethernet or Wi-Fi shields, and SD card readers, you can create a wide range of network-based solutions, all powered by a simple microcontroller.
Whether you're looking to monitor your home, store sensor data, or serve files remotely, these projects offer a great starting point. As you dive deeper into Arduino, you’ll discover even more ways to make your projects more connected and functional.
Happy building!